Data.Binary
AboutData.Binary is a library for high performance binary serialisation of Haskell data. It uses the ByteString library to achieve efficient, lazy reading and writing of structures in binary format. Chris Eidhof writes on his use of Data.Binary implementing a full-text search engine: "The communication with Sphinx is done using a quite low-level binary protocol, but Data.Binary saved the day: it made it very easy for us to parse all the binary things. Especially the use of the Get and Put monads are a big improvement over the manual reading and keeping track of positions, as is done in the PHP/Python clients." ExampleFor example, to serialise an interpreter's abstract syntax tree to binary format:import Data.Binary import Control.Monad import Codec.Compression.GZip -- A Haskell AST structure data Exp = IntE Int | OpE String Exp Exp deriving Eq -- An instance of Binary to encode and decode an Exp in binary instance Binary Exp where put (IntE i) = put (0 :: Word8) >> put i put (OpE s e1 e2) = put (1 :: Word8) >> put s >> put e1 >> put e2 get = do tag <- getWord8 case tag of 0 -> liftM IntE get 1 -> liftM3 OpE get get get -- A test expression e = OpE "*" (IntE 7) (OpE "/" (IntE 4) (IntE 2)) -- Serialise and compress with gzip, then decompress and deserialise main = do let t = compress (encode e) print t let e' = decode (decompress t) print (e == e') Download
DownloadProject Activity![]() Starring...The Binary Strike Force
|
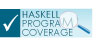
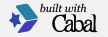
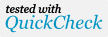